Integrating Air-Web
Step1.Get The Tokens
- BROWSER_ENCRYPTION_KEY(Browser Token):Used for redirection parameter
- MERCHANT_ENCRYPTION_KEY(Merchant Token):Used to verify payment notification
Parameter | Description |
---|---|
MERCHANT_ID | Please use the one indicated in Getting Started for testing purposes |
MERCHANTHASH | A SHA512 hash value generated by combining the Merchant hash key, Merchant ID, Settlement Type, Order ID and Amount. To create the merchant hash, please use the Development Merchant Hash Key indicated in Getting Started |
ORDER_ID | Order Identifier set by the merchant |
SESSION_ID | Session Identifier must be unique for each request call |
AMOUNT | Total price amount in Japanese Yen |
SETTLEMENT_TYPE | '00' = Not Specified; '01' = Credit Card; '02' = Convenience Stores (CVS) |
See API Parameters Page for further details.
Sample Code
<<Source>>/include/ConfirmActionManager.php
private $merchant = new MerchantInfo(); $data = new PurchaseData(); $ch = new HashCodeCreater(); $data->setMerchantId($data->getMerchantId()); $data->setOrderId($merchant->createOrderID()); $data->setSessionId($session_id()); $data->setAmount($form->getAmount()); $data->setSettlementType($form->getSettlementType); $hashCode = $ch->getHash($data->getMerchantId(), $data->getSettlementType(), $data->getOrderId(), $data->getAmount()); $data->setMerchantHash($hashCode); $purchaseData = $this->getPurchaseData($form); // HTTP POST $result = $this->sendPOST($purchaseData); return $result;
<<Source>>/jp/co/veritrans/vtweb/sample/server/action/ConfirmAction.java
Map settlementInfo = new LinkedHashMap(); MerchantConf merchantInfo = MerchantConf.getInfo(); settlementInfo.put("MERCHANT_ID", merchantInfo.getMerchantID()); HashCodeCreater4Merchant hash = new HashCodeCreater4Merchant(); settlementInfo.put("ORDER_ID", orderId); settlementInfo.put("SESSION_ID", sessionId); settlementInfo.put("AMOUNT", Integer.parseInt(form.getAmount())); settlementInfo.put("SETTLEMENT_TYPE", form.getSettlementType()); String hashCode = hash.getHash(merchantInfo.getMerchantID(), form.getSettlementType(), orderId, form.getAmount()); settlementInfo.put("MERCHANTHASH", hashCode); BufferedWriter bw = new BufferedWriter(new OutputStreamWriter(con.getOutputStream(), Constants.VTW_ENCODE)); String postData = getPostData(settlementInfo); //POST用に整形したデータを返却する。 - Generate Data in POST format bw.write(postData); bw.flush(); bw.close(); BufferedReader in = new BufferedReader(new InputStreamReader(con.getInputStream(), Constants.VTW_ENCODE)); EncryptionKey ret = new EncryptionKey(); String line; try { while ((line = in.readLine()) != null) { if (line.startsWith("MERCHANT_ENCRYPTION_KEY")) { String tmp = line.substring(24); registKey(orderId, tmp, sessionId); ret.setMerchantEncryptionKey(tmp); } else if (line.startsWith("BROWSER_ENCRYPTION_KEY")) { String tmp = line.substring(23); ret.setBrowserEncryptionKey(tmp); } else if (line.startsWith("ERROR_MESSAGE")) { String tmp = URLDecoder.decode(line.substring(14), Constants.VTW_ENCODE); ret.setErrorMessage(tmp); } } return ret; } catch (Throwable th) { return null; }
Step2.Creating the Form for Redirection
Air-Web Payment Page Screenshots
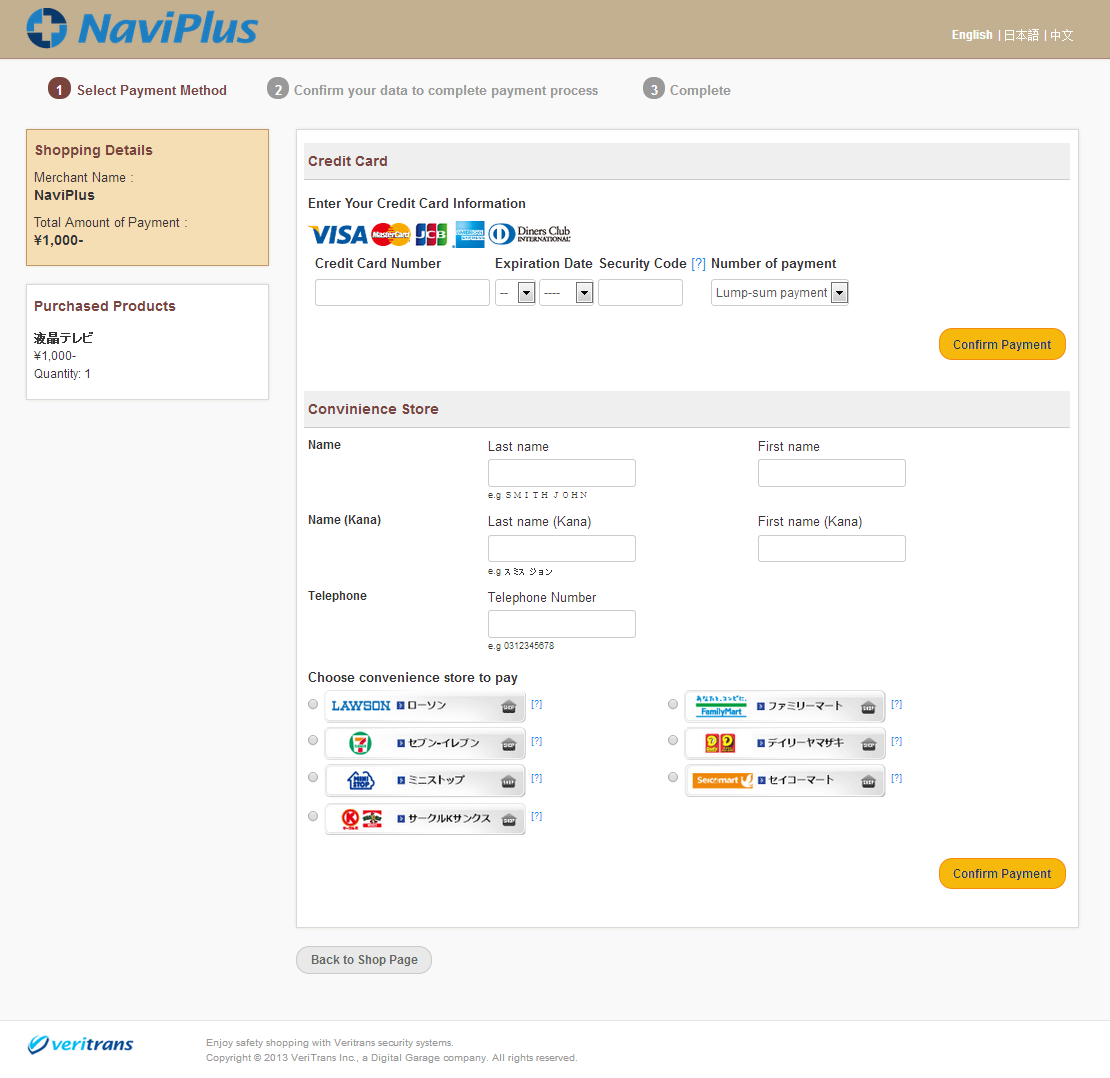
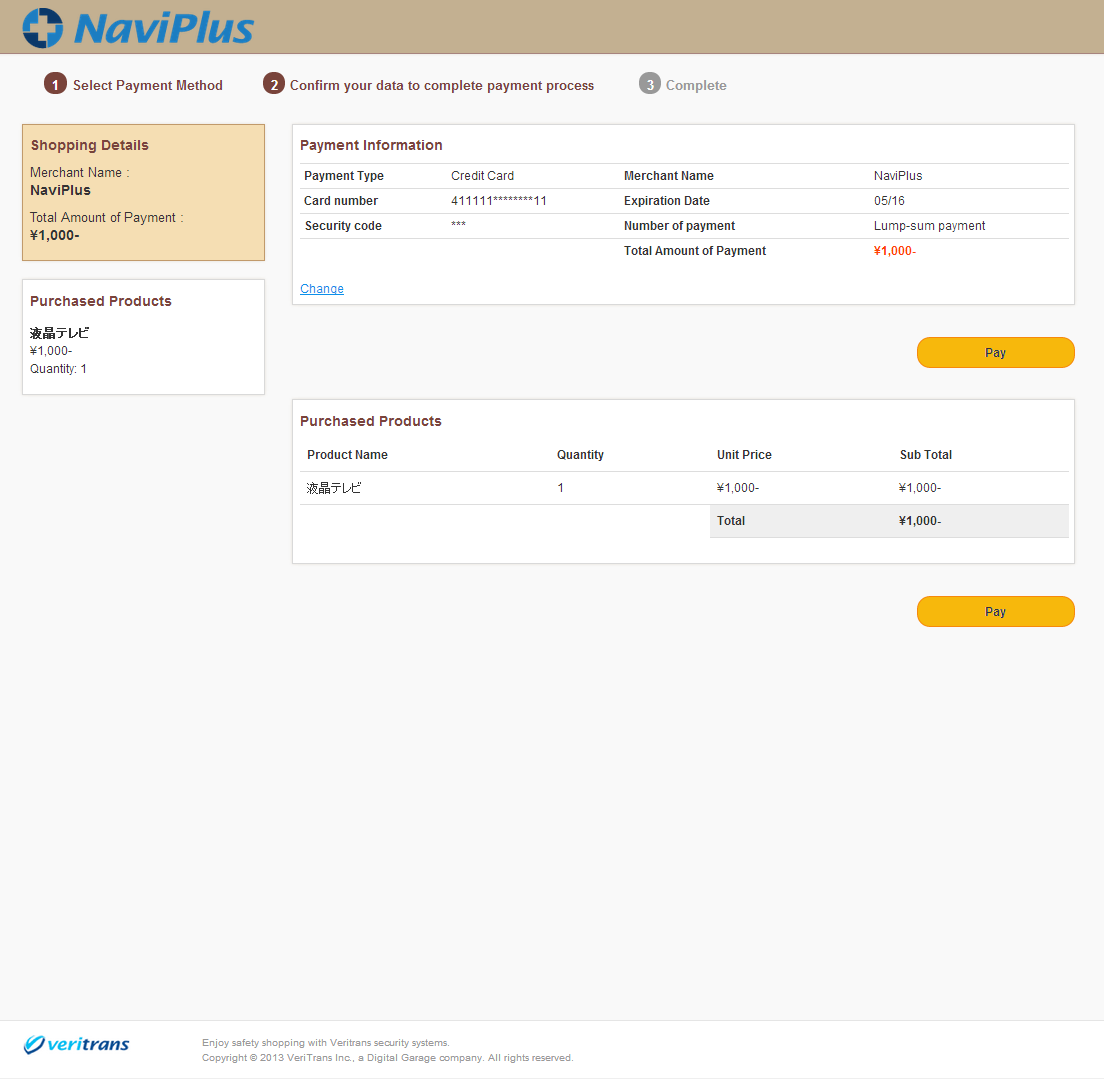
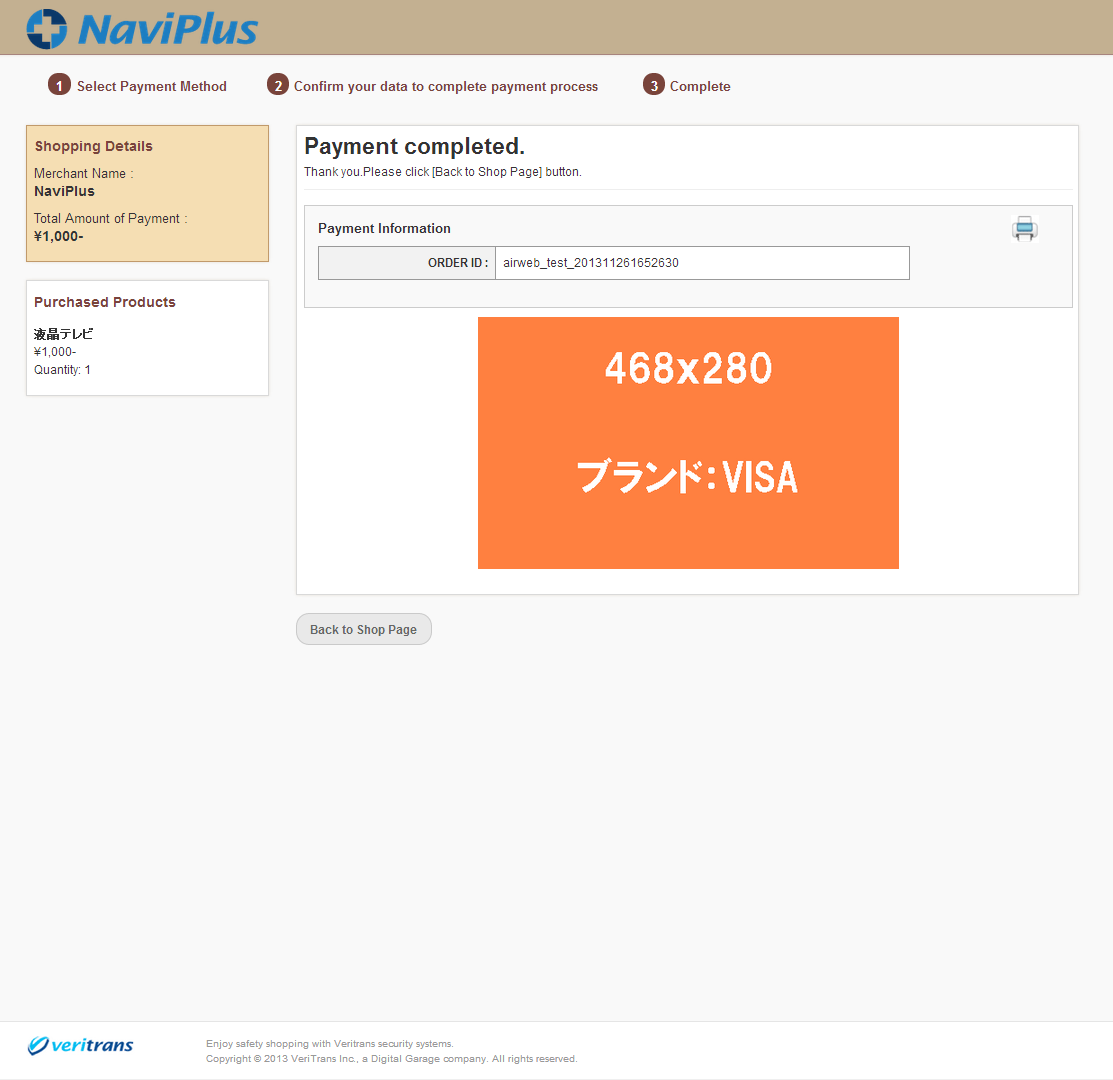
Sample Code
<<Source>>/sample/jump.php
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01//EN" "http://www.w3.org/TR/html4/strict.dtd"> <html lang="ja"> <head> <?php include("../include_head.php"); ?> <title><?php echo $lang['JUMP.TITLE'];?></title> </head> <body> <div class="contents"> <h1 align="center"><?php echo $lang['JUMP.HEADER'];?></h1> <form action="<?= PAYMENT_URL ?>" method="post" onSubmit="document.getElementById('submitBtn').disabled=true;"> <input type="hidden" name="MERCHANT_ID" value="<?= $merchant->getMerchantID() ?>" /> <input type="hidden" name="ORDER_ID" value="<?= $merchant->getOrderID() ?>" /> <input type="hidden" name="BROWSER_ENCRYPTION_KEY" value="<?= $merchant->getSettlementKey1A() ?>" /> <input id="submitBtn" type="submit" value="submit" /> </form> </div> </body> </html>
<<Source>>/jsp/self/jump.jsp
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01//EN" "http://www.w3.org/TR/html4/strict.dtd"> <html lang="ja"> <head> <%@ include file="include_head.jsp" %> <title>お支払手続きへ</title> <% MerchantConf info = MerchantConf.getInfo(); String sessionID = session.getId(); String key1a = (String)request.getAttribute("SETTLEMENT_KEY1A"); String merchantId = (String)request.getAttribute("MERCHANT_ID"); String orderId = (String)request.getAttribute("ORDER_ID"); %> </head> <body> <div class="contents"> <h1 align="center">お支払手続きへ</h1> <form action="<%= info.getVtWebSettlementUrl() %>" method="post" onsubmit="document.getElementById('submitBtn').disabled=true;"> <input type="hidden" name="MERCHANT_ID" value="<%= merchantId %>" /> <input type="hidden" name="ORDER_ID" value="<%= orderId %>" /> <input type="hidden" name="BROWSER_ENCRYPTION_KEY" value="<%= key1a %>" /> <input id="submitBtn" type="submit" value="submit" /> </form> </div> </body> </html>
Step3.Handling Response
The merchant needs to verify every notification request. The parameters that will be verified are ORDER_ID and MERCHANT_ENCRYPTION_KEY. Please make sure that both parameters match with the ones inside your merchant database.
Sample Code
<<Source>>/sample/DoPostActionAW.php
// AWからのRequestの内容確認 - Validate request's content $result = $KeyValidate->resultCheck(); // チェック結果を表示 header("text/html; charset=" . AW_HTML_ENCODE); if ($result) { $KeyValidate->updateKeyBox(); echo "OK\n"; } else { echo "ERR\n"; }
<<Source>>/jp/co/veritrans/airweb/sample/server/DoPostActionAW.java
ResultDataBean databean = new ResultDataBean(); // リクエストをマップに格納 - Validate request's content databean.setOrderId(orderId); databean.setmStatus(URLDecoder.decode(request.getParameter(Constants.AWRES_M_STATUS), Constants.AW_ENCODE)); databean.setmErrMsg(URLDecoder.decode(request.getParameter(Constants.AWRES_M_ERR_MSG), Constants.AW_ENCODE)); databean.setvResultCode(URLDecoder.decode(request.getParameter(Constants.AWRES_V_RESULT_CODE), Constants.AW_ENCODE)); ResultCheck rcheck = new ResultCheck(); boolean checkflag; checkflag = rcheck.resultCheck(databean); // AWへ返信 - Return result if (checkflag) { key.put(Constants.AWRES_M_STATUS, databean.getmStatus()); key.put(Constants.AWRES_M_ERR_MSG, databean.getmErrMsg()); key.put(Constants.AWRES_V_RESULT_CODE, databean.getvResultCode()); keyBox.setBox(orderId, key); response.setContentType(CONTENT_TYPE); ServletOutputStream out = response.getOutputStream(); out.println("OK"); } else { response.setContentType(CONTENT_TYPE); ServletOutputStream out = response.getOutputStream(); out.println("ERR"); }
- Prev:< [Getting Started]
- Next:[Testing Air-Web] >